Coffee Machine (Object Oriented Programming)
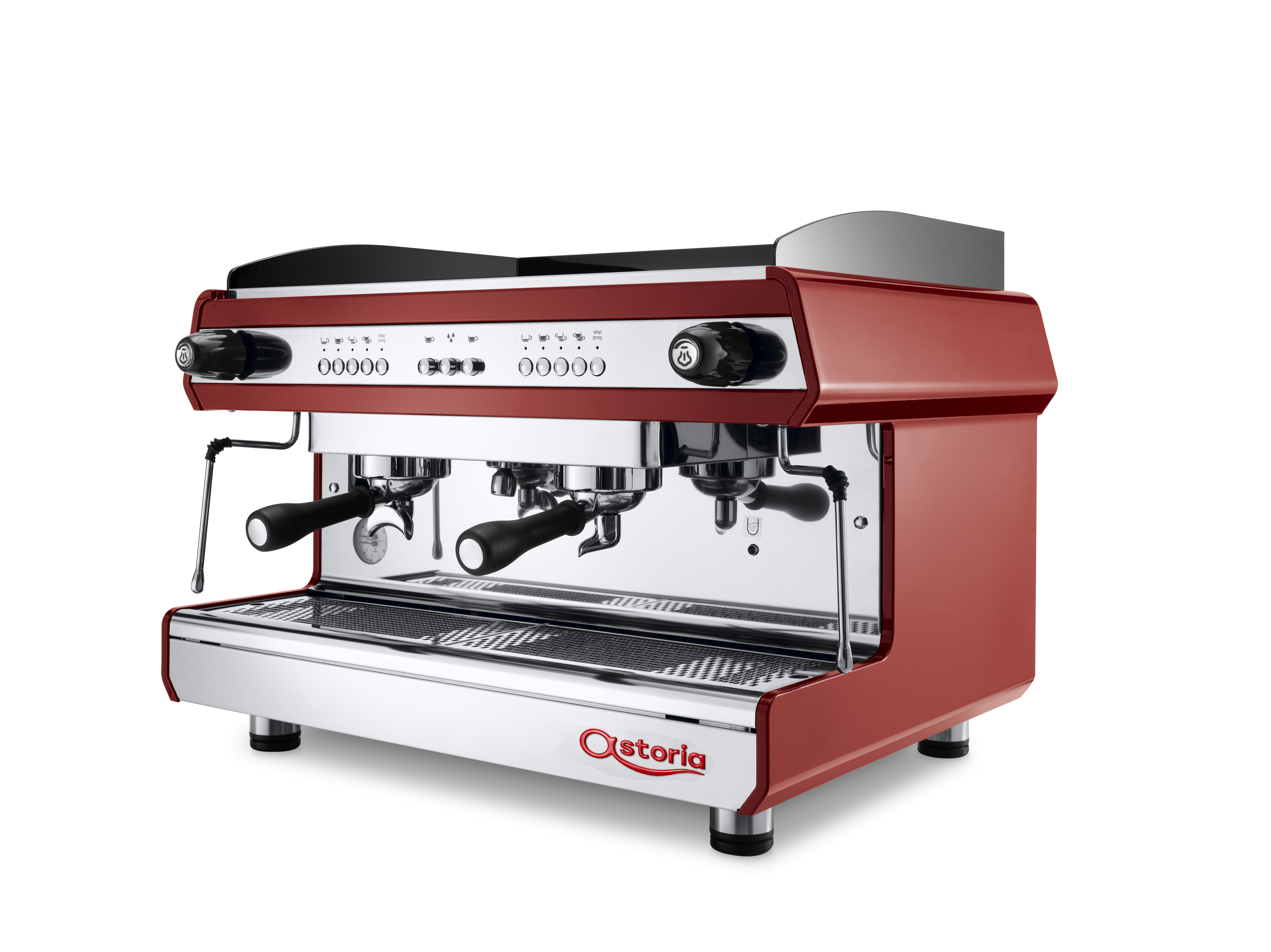
Coffee Machine Simulator in Python
Hey coffee lovers,
I recently created a Coffee Machine Simulator using Python to practice object-oriented programming concepts. This project simulates a coffee machine where users can select their favorite coffee, check resources, and handle payments. It's an interactive project that ties together several Python modules to give a functional user experience. Let's dive into how it works!
Project Overview
The Coffee Machine Simulator is built using custom Python classes, including Menu, MenuItem, CoffeeMaker, and MoneyMachine. The project allows users to interact with a virtual coffee machine, order drinks, and manage resources and payments. It's an excellent way to practice object-oriented programming (OOP) principles.
Key Features
Menu System: The project provides a menu of available drinks, allowing users to choose their preferred coffee.
Resource Management: The CoffeeMaker class checks if there are sufficient ingredients to make the selected drink and updates the machine's resources accordingly.
Payment Handling: Using the MoneyMachine class, the project calculates the cost of the drink and processes the payment, simulating a real-world vending machine experience.
Reports: Users can request reports to check the machine's available resources and the total earnings.
Step-by-Step Breakdown
1. Setting Up the Classes
The project is built using three main classes: Menu, CoffeeMaker, and MoneyMachine. The Menu class provides the list of available drinks, CoffeeMaker manages the coffee machine's resources, and MoneyMachine handles all payment-related functions.
2. The Menu System
The user is presented with a list of drink options: latte, espresso, or cappuccino. They can select one, request a report, or turn off the machine. The program waits for user input and responds accordingly.
3. Resource Management
The CoffeeMaker class checks if the coffee machine has enough resources (water, milk, coffee) to make the selected drink. If resources are sufficient, it makes the coffee; if not, it informs the user.
4. Payment Processing
The MoneyMachine class calculates the cost of the selected drink and prompts the user to insert the correct amount of money. If the payment is sufficient, the machine processes the order; otherwise, it refunds the money.
5. Reports
Users can type report
to get a report of the machine's current resources and total money earned so far.
Python Skills Utilized
Object-Oriented Programming: The project is structured around OOP principles, utilizing classes and methods to simulate the coffee machine's behavior.
Resource Management: The CoffeeMaker class helps manage and track resources like water, milk, and coffee, adjusting quantities based on user choices.
Payment Handling: Using the MoneyMachine class, the project simulates real-world payments, ensuring the right amount is inserted before processing the coffee order.
Real-World Use Cases
This project could be extended to simulate other types of vending machines, such as snack dispensers or juice machines.
Develop a more complex system to manage larger inventories or add more detailed pricing options and product lists.
Use the payment system as a starting point for developing small e-commerce applications or point-of-sale systems.
Conclusion
The Coffee Machine Simulator is an engaging way to practice OOP, resource management, and payment handling in Python. It’s a simple yet powerful example of how classes and objects can be used to simulate real-world systems.
You can check out the full code for this project on my GitHub repository and try it out yourself!
Stay tuned for more Python projects coming soon!
Watch the demo video here:
Category: projects
306
2
Comments